We love JavaScript. It's mature, it moves fast, and its developer community is vibrant and passionate. It's awesome. But it is always tiring to wait for npm start
to actually start. Be it a simple node server, or a react app, it always gives us time to get a coffee for ourselves.
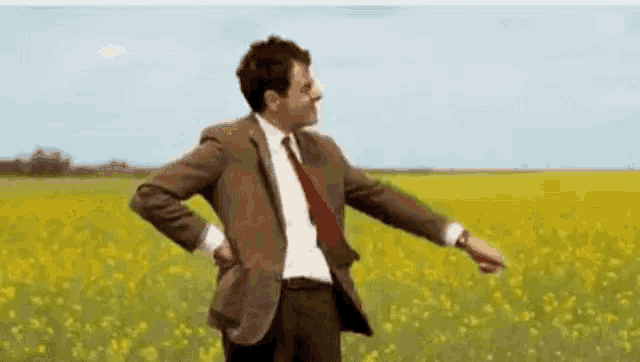
Bun is a result of a developer asking this question: "Why should I wait long for a simple server to start?" So they've made it lighter, faster and flexible than node. You can do anything that you do with npm, pnpm, yarn or jest.
What is Bun?
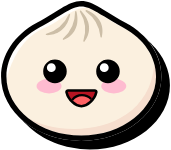
At its core, Bun is a JavaScript runtime environment that allows you to run JavaScript code on the server-side. Where Bun really shines is in its flexibility and ease of use. It was created by the same team that developed the popular ORM library Sequelize, and it's designed to be a more flexible and modular alternative to Node.js.
One of the key features of Bun is its modular architecture. Unlike Node.js, which requires you to install a lot of different packages and dependencies to get started, Bun comes with everything you need built-in. This makes it much easier to get started with Bun and reduces the risk of dependency conflicts.
Bun's goal is simple: eliminate slowness and complexity without throwing away everything that's great about JavaScript. Your favorite libraries and frameworks should still work, and you shouldn't need to unlearn the conventions you're familiar with.
Features
Package Manager
The bun
CLI contains a Node.js-compatible package manager designed to be a dramatically faster replacement for npm
, yarn
, and pnpm
. It's a standalone tool that will work in pre-existing Node.js projects; if your project has a package.json
, bun install
can help you speed up your workflow.
bun install
bun add <package> [--dev|--production|--peer]
bun remove <package>
bun update <package>
Speed
One of the main advantages of Bun over Node.js is its speed. Bun claims to be up to 10 times faster than Node.js in some benchmarks.
Installing new packages in Bun is 17x faster than any runtime environments available till date
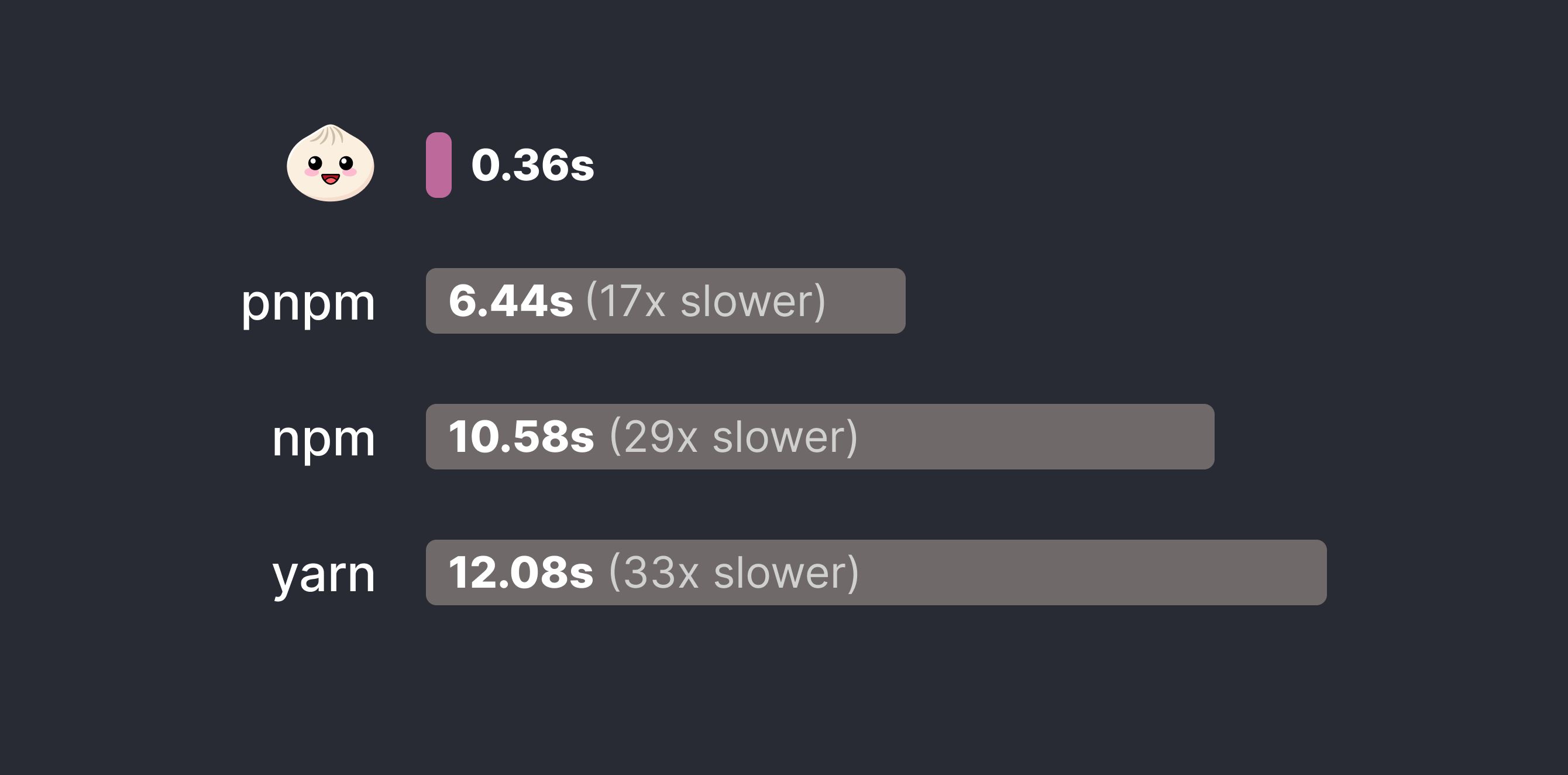
Bun is fast, starting up to 4x faster than Node.js. This difference is only magnified when running a TypeScript file, which requires transpilation before it can be run by Node.js.
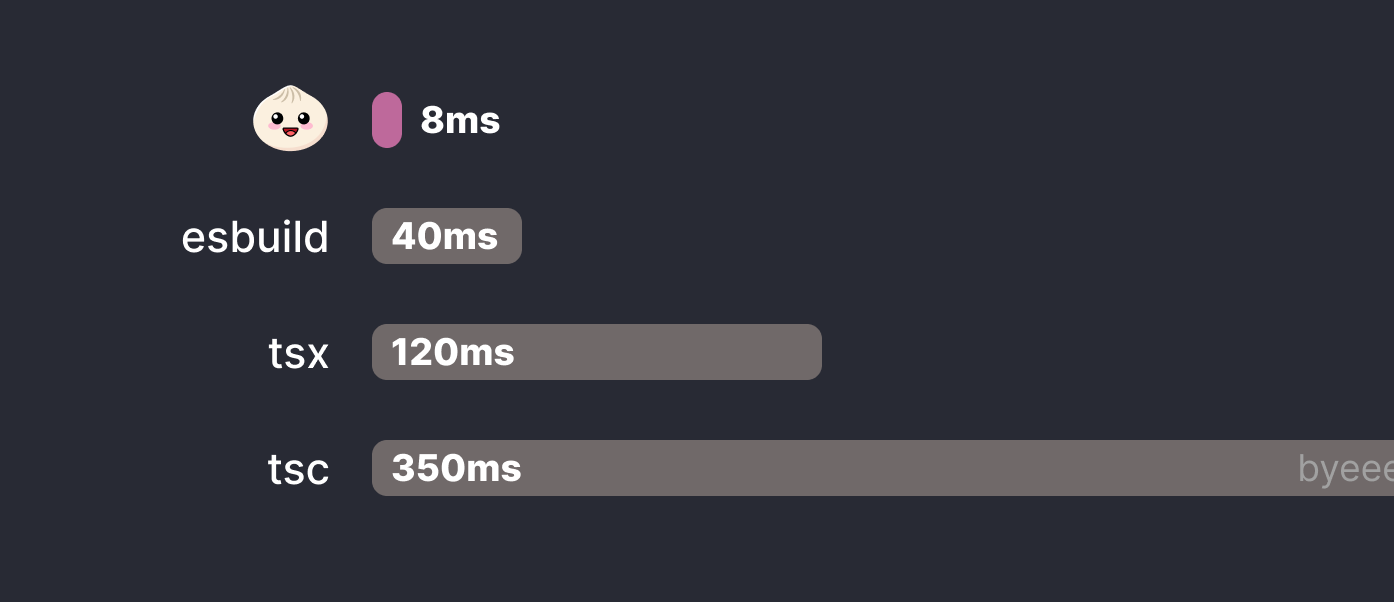
This doesn't stop here. Bun beats the rest of the runtime environments in all aspects including building, starting development servers, testing and even code minification.
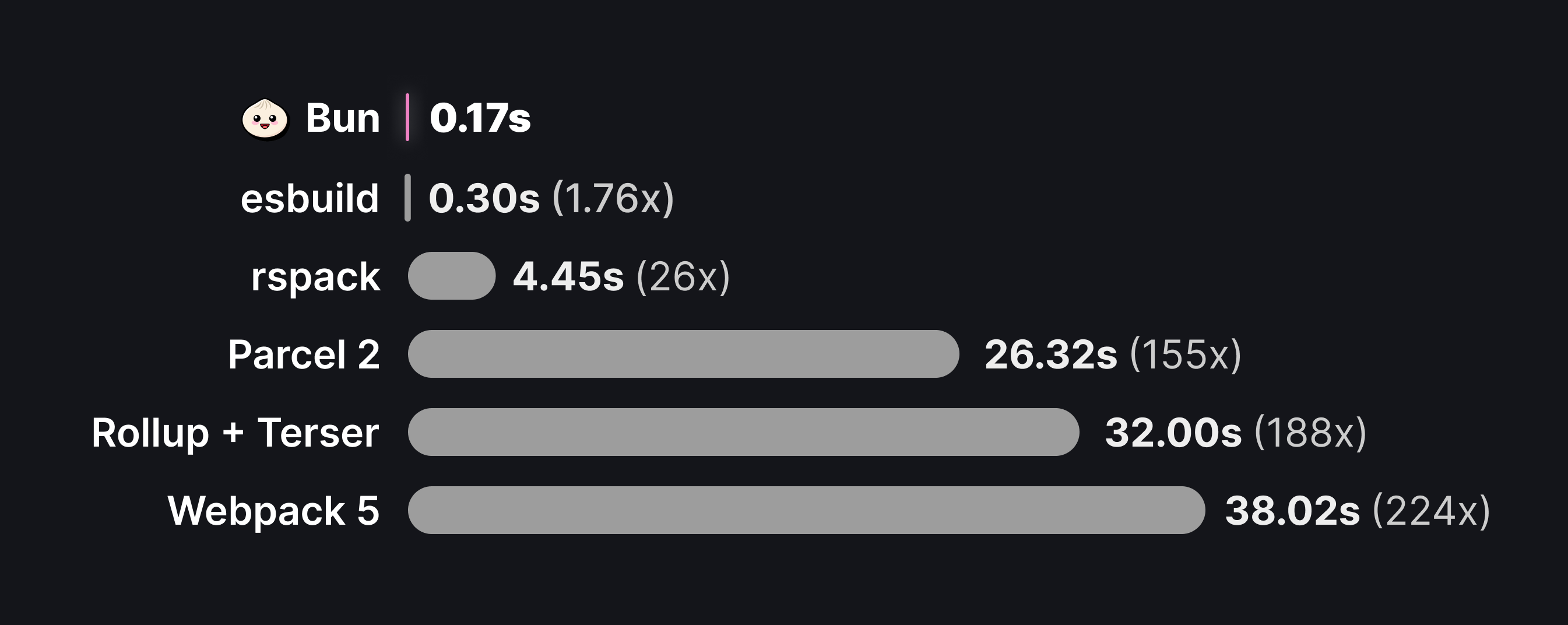
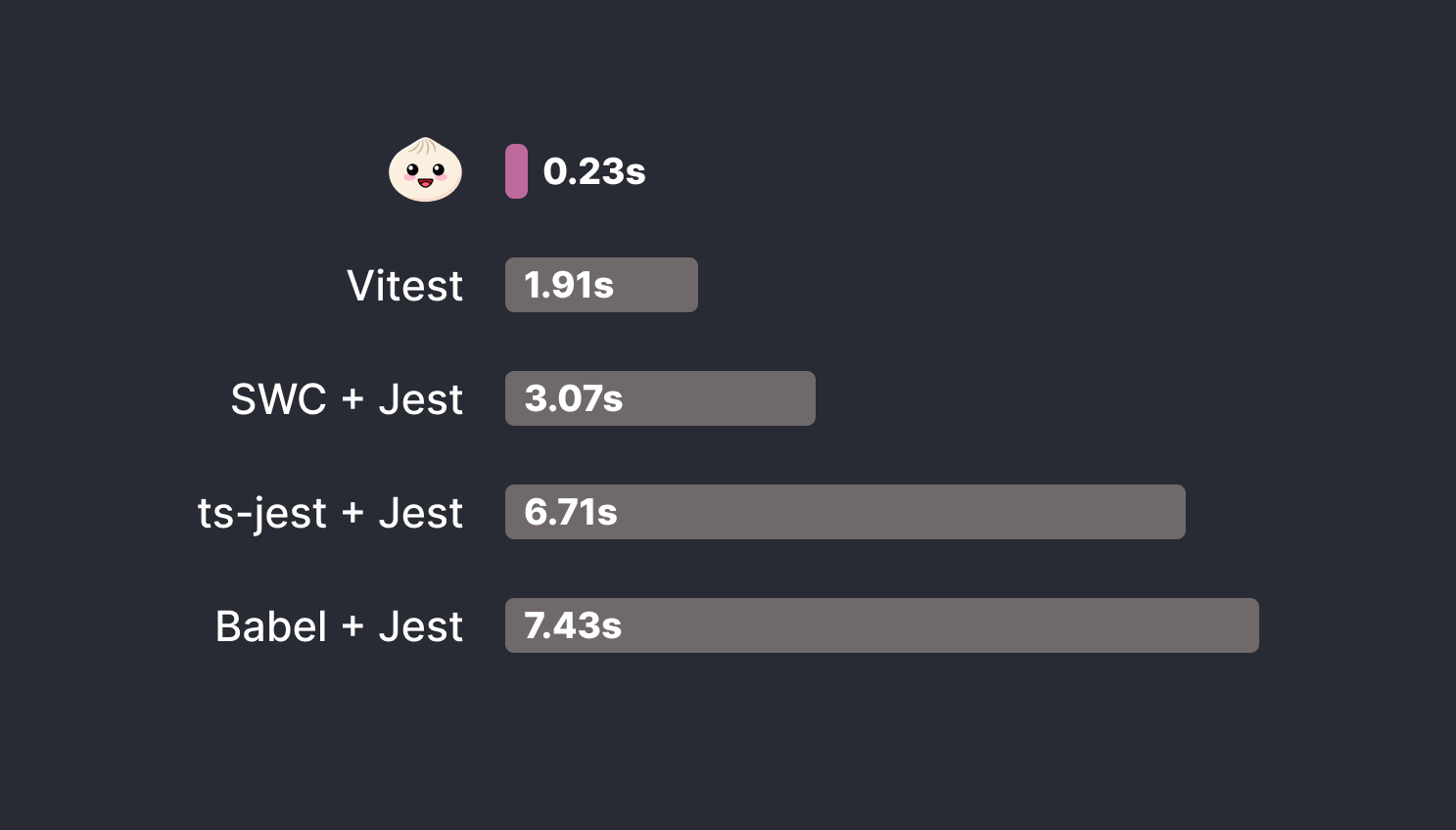
How does it achieve this impressive performance?
The answer lies in the design choices of Bun. Bun uses a different JavaScript engine than Node JS. Node JS and other runtimes are build using Google's V8 engine which is used in Chrome based web browsers. But Bun uses Apple's Webkit engine which powers the Safari browser.
Learn more about different web browser engines here
Elegant APIs
HTTP Server
Start an HTTP server in Bun with Bun.serve
Bun.serve({
port: 8000,
fetch(req) {
const url = new URL(req.url);
if (url.pathname === "/") return new Response("Home page!");
if (url.pathname === "/blog") return new Response("Blog!");
return new Response("404!");
}
})
You can also create websockets with Bun.serve()
.
Bun.serve({
fetch(req, server) {}, // upgrade logic
websocket: {
message(ws, message) {}, // a message is received
open(ws) {}, // a socket is opened
close(ws, code, message) {}, // a socket is closed
drain(ws) {}, // the socket is ready to receive more data
},
})
File Handling
Handle files with BunFile
instance more easily with Bun.file()
API. A BunFile
represents a lazily-loaded file; initializing it does not actually read the file from disk.
const foo = Bun.file("filename.txt");
TCP Connections
Creating TCP connections is now easier with Bun. To create a TCP connection, use Bun.listen()
Bun.listen({
hostname: "localhost",
port: 8080,
socket: {
data(socket, data) {}, // message received from client
open(socket) {}, // socket opened
close(socket) {}, // socket closed
drain(socket) {}, // socket ready for more data
error(socket, error) {}, // error handler
},
});
Similarly you can connect to a TCP connection with Bun.connect
. You can also enforce TLS with tls: true
.
SQLite3 Database
Bun natively implements a high-performance SQLite3 driver. To use it import from the built-in bun:sqlite
module. The API is inspired by better-sqlite3
, but is written in native code to be faster.
import { Database } from "bun:sqlite";
const db = new Database(":memory:");
const query = db.query("select 'Bun' as runtime;");
query.get(); // => { runtime: "Bun" }
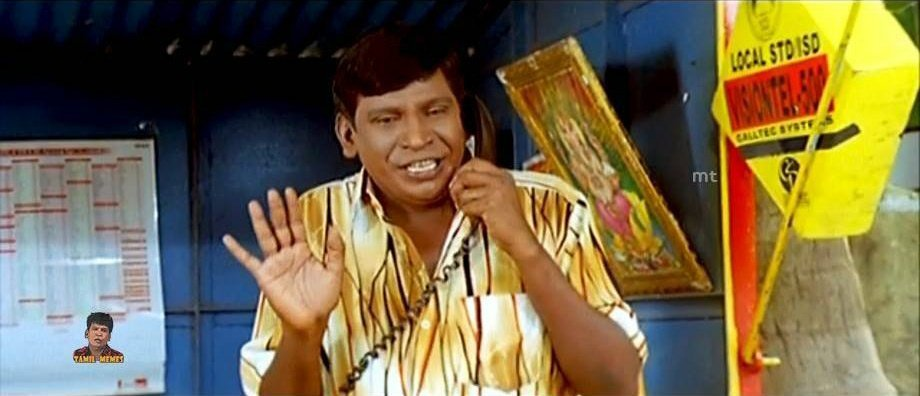
Install Bun today!
# cURL
curl -fsSL https://bun.sh/install | bash
# npm
npm install -g bun
# brew
brew tap oven-sh/bun
brew install bun
# docker
docker pull oven/bun
docker run --rm --init --ulimit memlock=-1:-1 oven/bun
Resources
- Bun - https://bun.sh
- Documentation - https://bun.sh/docs